What is the difference between var and let in JavaScript?
Variable declaration in JavaScript blocks can sometimes be confusing especially within complicated scopes. This minipost aims to clarify the difference between var
and let
JavaScript keyword for variable declarations.
In JavaScript, function
is the only thing that create a new scope. Therefore, the same variable declaration in a global or a local scope yields different outputs. In JavaScript, blocks are used to group the body of an if
statement or a loop. However, you are allowed to use free-standing blocks(blocks outside an if statement or a loop).
For the simplicity of the examples below and rather than using polluted code with if statements and loops we will demonstrate the case using free-standing blocks:
var x = 1; { var x = 2; // Same variable console.log(x); // Output: 2 } console.log(x); // Output: 2
The variable x
inside the block refers to the same variable as the one outside the block.
The let
keyword works like the var
keyword but it creates a variable that is local to the enclosing block and not to the enclosing function. Therefore, the previous example will change by using the let
keyword inside the free standing block and creating a local scope within the block only.
var x = 1; { let x = 2; // Different variable console.log(x); // Output: 2 } console.log(x); // Output: 1
The keyword let
allows the variable declaration with limited scope to the block, statement or expression on which it is used. Unlikely, the var
keyword declares a variable globally/locally to an entire function, regardless of block scope.
Global Scoping Rules
Another difference between var
/ let
appears at the top-level declarations. At the top level of programs and functions let
, unlike var
does not create a property on the global object.
var x = 1; let y = 1; console.log(this.x); // Output: 1 console.log(this.y); // Output: undefined
Redeclarations
A typical redeclaration in JavaScript with var
keyword can be performed with the following way without any problems:
var x = 1; var x = 2;
However, variable redeclaration with let
keyword throws a SyntaxError
, stating that the variable has already been defined:
let x = 1; let x = 2;
throws the exception:
let x = 2; ^ SyntaxError: Identifier 'x' has already been declared at new Script (vm.js:79:7) at createScript (vm.js:251:10) at Object.runInThisContext (vm.js:303:10) at Module._compile (internal/modules/cjs/loader.js:657:28) at Object.Module._extensions..js (internal/modules/cjs/loader.js:700:10) at Module.load (internal/modules/cjs/loader.js:599:32) at tryModuleLoad (internal/modules/cjs/loader.js:538:12) at Function.Module._load (internal/modules/cjs/loader.js:530:3) at Function.Module.runMain (internal/modules/cjs/loader.js:742:12) at startup (internal/bootstrap/node.js:279:19)
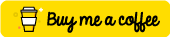