Tricky value comparisons with abstract and strict equality operator in JavaScript
Comparisons with double equations in JavaScript can easily lead to cases where the evaluation of a comparison is not so straight forward. This minipost presents a collection of such cases that require developer attention.
Value Equality comparisons
JavaScript supports two types of equality comparisons:
- The abstract / type converting comparison with the equality operator(
==
) - The strict comparison with the identity / strict equality operator(
===
)
In the abstract comparison(==
) JavaScript uses Automatic Type Conversion to convert the operands to the same type prior comparing them. However, in the strict comparison(===
) the output is true if and only if both operands are of the same type and their contents match.
console.log(0 == false); // Output: true console.log(0 === false); // Output: false
In this expression 0
is being booleanized as false
and the expression returns true
.
console.log("" == false); // Output: true console.log("" === false); // Output: false console.log(NaN == false); // Output: false console.log(NaN === false); // Output: false
The rules for converting Strings and Numbers to Boolean values in JavaScript state that 0
, NaN
and the empty String (""
) are evaluated as false
.
console.log(null == undefined); // Output: true console.log(null === undefined); // Output: false
null
and undefined
should be treated as interchangeable. Therefore, their comparisons evaluate to true
.
console.log(1 == "1"); // Output: true console.log(1 === "1"); // Output: false
console.log(0 == null); // Output: false console.log(0 === null); // Output: false
In a comparison when null
or undefined
appears on either side of the operator it produces true if both sides are null
/ undefined
.
console.log(NaN == NaN); // Output: false console.log(NaN === NaN); // Output: false
In JavaScript the only value that is not equal to itself is NaN
. To clarify this, NaN
is supposed to denote the result of a nonsensical computation, and as such a result is not equal to the result of any other nonsensical computation.
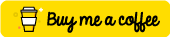